Learn Multi platform eZ80 Assembly
Programming... For more power!
Platform Specific lessons
These lessons cover the platform specific tasks required for programming
eZ80 systems.
Lesson
P1 - Bitmap Hello World and sprite drawing on the Ti-84 in
16bpp
Lets take a look at basic graphics tasks on the TI-84+CE.
We'll code up a text drawing routine, and show our 'Chibiko
mascot to the screen.
|
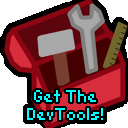 |
hello.asm
|
|
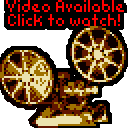 |
Bitmap Drawing
We'll need a bitmap graphic for today's example!
Each pixel uses two bytes, in the format %RRRRRGGGGGBBBBB-
You can export data in the correct byte format with my Akusprite
Editor.
|
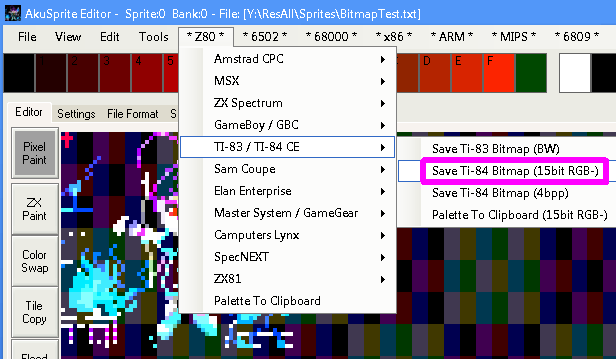 |
The Screen is 320*240 pixels - and by default it's in 16bpp mode,
meaning each pixel takes 2 bytes.
VRAM starts from memory address $D40000 so our formula for the VRAM
destination is:
;VRAM=$D40000 + Ypos*320*2 + Xpos*2
Our sample sprite is 58x54 pixels
To draw our sprite we just transfer the bytes from our source bitmap
to screen memory.
After each line we add 640 (320 *2) to move down a line and repeat.
|
|
Here is the result!
We drew our 'Chibiko' mascot at the top right of the screen. |
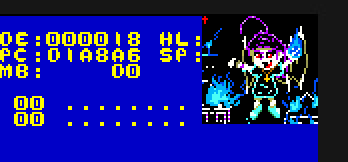 |
If we want to clear our screen, we can do so by writing bytes to
the screen ram.
Here we're filling the screen a darkish blue.
This example writes two bytes at a time, so BC doesn't go over $FFFF |
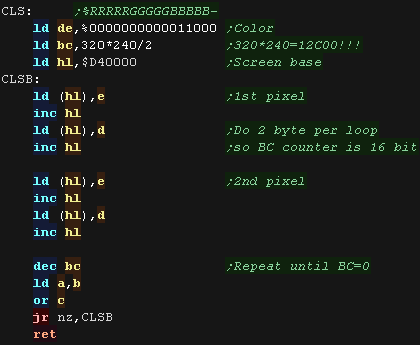 |
Bitmap Font Character Drawing
We're going to use a 1 bit per pixel font (1bpp - black and
white), but our screen is 16bpp, so we'll have to convert the bits
of our font to pixel words to color our screen. |
 |
We're going to need a GetScreenPos function to
calculate the VRAM Destination for the next character from a cursor
X and Y
We'll use this to calculate the starting position of our character.
We also have a GetNextLine function to move down
one pixel line - we'll use this every line of our font.
|
|
We need a multiply routine for our function.
This one is super basic, but it will suffice for our needs.
|
|
Our PrintChar routine will need to calculate the source address of
the font data... each character in our font is 8 lines - so 8 bytes.
Our font also has no characters before space.
We use our GetScreenPos to calculate the VRAM destination
|
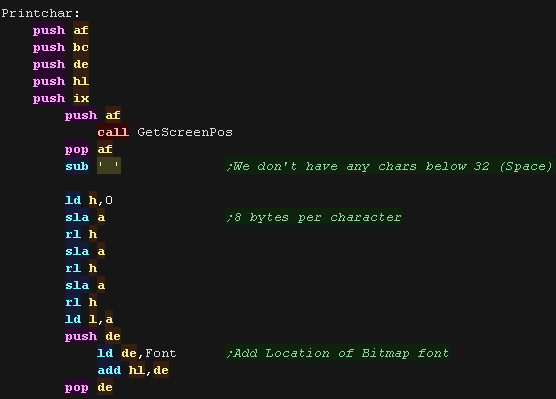 |
We load a byte from our font into the C register for each line
We then move one bit at a time from C, and either draw the blue
background if the bit is 0, or yellow forground if the bit is 1.
We repeat for each line of our font, then move the Xpos by 1 to move
across the screen.
|
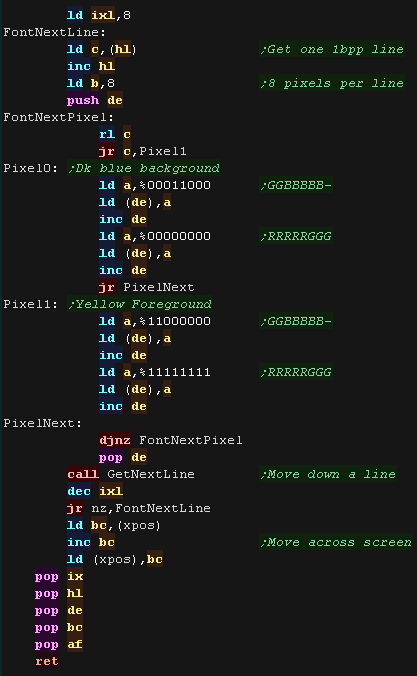 |
Our font will be shown to the screen.
We can extend this PrintChar routine into a PrintString as required. |
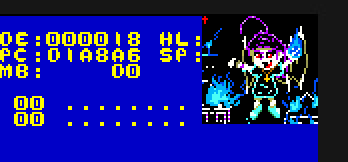 |
We
looked at 16bpp this time, but those big characters will eat up
our program memory.
Alternatively we can use the much more memory efficient 4bpp -
16 color mode... but we'll look at that next time!
|
 |
Lesson
P2 - Bitmap Hello World and sprite drawing on the Ti-84 in
16 colors (4bpp)
Lets take a look at basic graphics tasks on the TI-84+CE.
We'll code up a text drawing routine, and show our 'Chibiko.
mascot to the screen.... this time in 16 colors!
|
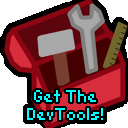 |
4bpptest.asm
|
|
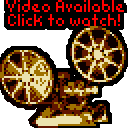 |
 |
We're going to use 16
color mode this time... while the palette is still defined in 15
bits, this will allow us to use smaller image files, saving memory
on our bitmap graphics |
Bitmap Drawing
We'll need a bitmap graphic for today's example!
Each byte has two pixels, but strangely the Leftmost pixel is in
the bottom nibble, and the Rightmost is in the top, so the format
of a LR pair is %RRRRLLLL
You can export data in the correct byte
format for the sprite with my Akusprite Editor.... we
can also export the palette.
|
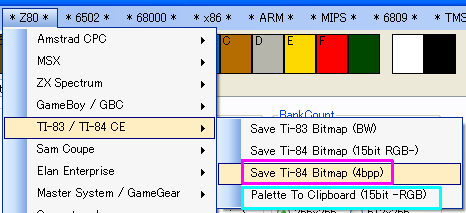 |
First we need to set up our screen, we do this with port
$E30018. We write two 'mode bits' Bits 1-3 set the screen mode, we
probably want to keep the other bits the same.
In this example We use mode 2 (4bpp - 16 color)
We also need to set up the palette. The colors are defined by
ports $E30200+ - each color is defined by 2 bytes in the
format %-RRRRRGGGGGBBBBB
|
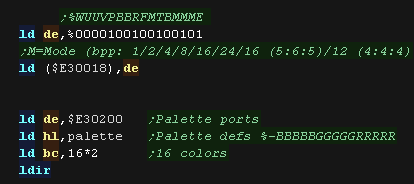
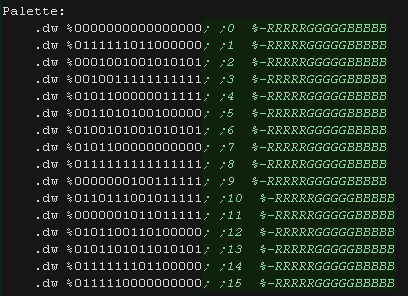 |
The Screen is 320*240 pixels - and each byte contains 2 pixels,
meaning each line is 160 bytes
VRAM starts from memory address $D40000 so our formula for the
VRAM destination is:
;VRAM=$D40000 + Ypos*320/2 + Xpos/2
Our sample sprite is 58x54 pixels
To draw our sprite we just transfer the bytes from our source
bitmap to screen memory.
After each line we add 160 (320 /2) to move down a line and
repeat.
|
|
Here is the result!
We drew our 'Chibiko' mascot at the top right of the screen.
We didn't clear the screen, so there's some corruption in the
background. |
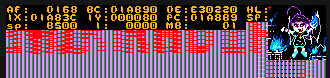 |
Before we return to the OS, we need to reset the screen to 16bpp
mode, otherwise the OS will not be able to show it's graphics
correctly, and the screen will be corrupted. |
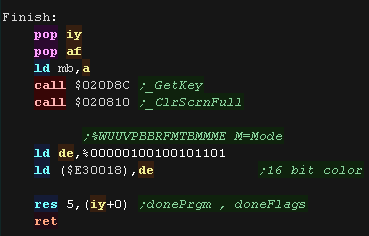 |
Bitmap Font Character Drawing
We're going to use a 1 bit per pixel font (1bpp - black and
white), but our screen is 16bpp, so we'll have to convert the bits
of our font to pixel words to color our screen. |
 |
We're going to need a GetScreenPos function to
calculate the VRAM Destination for the next character from a
cursor X and Y
We'll use this to calculate the starting position of our
character.
We also have a GetNextLine function to move
down one pixel line - we'll use this every line of our font.
|
|
We need a multiply routine for our function.
This one is super basic, but it will suffice for our needs.
|
|
Our PrintChar routine will need to calculate the source address
of the font data... each character in our font is 8 lines - so 8
bytes.
Our font also has no characters before space.
We use our GetScreenPos to calculate the VRAM destination
|
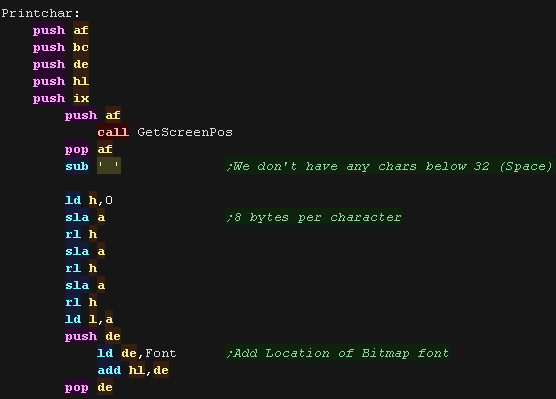 |
We load a byte from our font into the C register for each line
We then move two bits from C, and move them into the correct
positions for color 1 of our screen pixels.
Because we need to reverse the positions of the bits, we rotate
them out of C to the right, and into A on the left.
we repeat 4 times for each pixel of our screen.
|
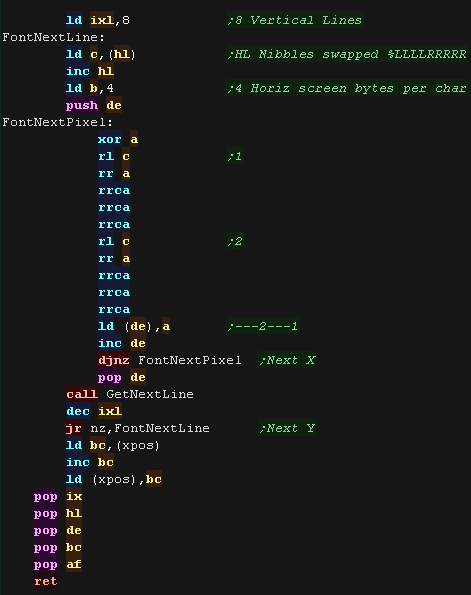 |
Our font will be shown to the screen.
We can extend this PrintChar routine into a PrintString as
required. |
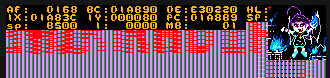 |
|