Learn Multi platform
65816 Assembly Programming... For
Monsters!
Simple
Samples

Drawing a bitmap
We're going to move a smiley around the screen with the joypad! |
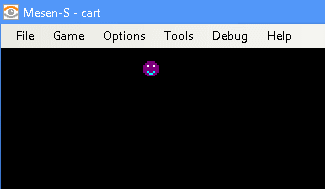 |
WLADX needs us to define the layout of our memory. We're defining
a simple single block of memory $20000 in size. |
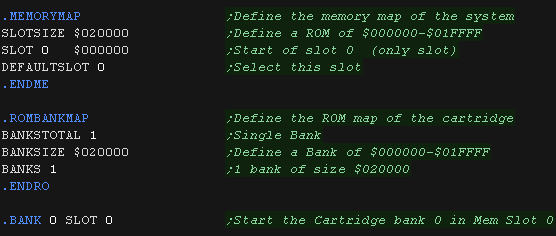 |
We define some direct page entries for our work.
Z_HL is made up of 3 bytes U, H and L for 24 bit addresses. |
 |
Our cartridge starts at address $8000.
We begin by stopping interrupts, enabling 65816 mode, and setting
the X/Y registers to 16 bit.
The Accumulator and memory functions are still 8 bit. |
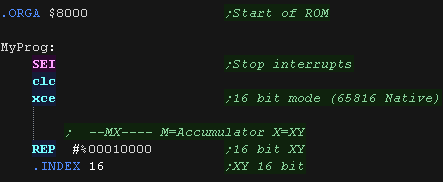 |
We need to set up our screen!
Unfortunately there's a lot of strange settings we need to set to do
this, but we've got basic values here to set up a screen with a
32x32 tilemap. |
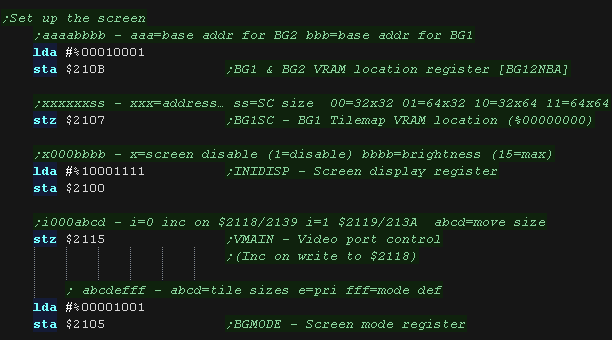 |
We need to set up the colors for our screen.
Color 0 is black
Color 1 is purple
Color 2 is cyan
Color 3 is white
|
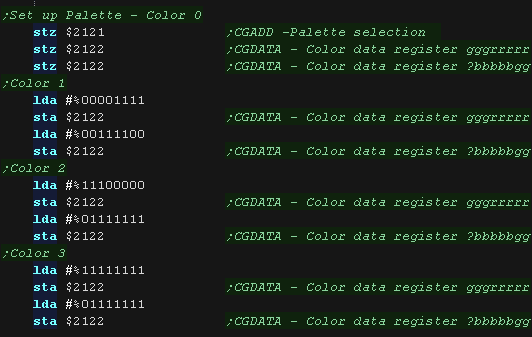 |
We define two tile patterns, our smiley and a blank tile to remove
it!
|
|
We need to transfer our two tiles into pattern RAM.
We load the source data into z_HL, and select the pattern address
$1000 as the destination with ports $2116/7
We transfer our pattern data (32 bytes per tile) using ports $2119/8
|
|
We need to set the scroll position of the tilemap, and zero all
the tiles in the tilemap.
The tilemap is 32x32, so there are $400 entries, and each one takes
2 bytes.
|
|
Now all our data has been transferred, we can turn
on our screen.
|
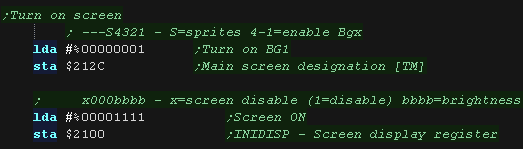 |
Tile 0 is our blank tile,
Tile 1 is our smiley.
We now need to calculate the VRAM address.
The tilemap is at VRAM address $0000 onwards, and each line is 32
tiles, so the formula is 'VRAM = (Ypos*32) + Xpos'.
We calculate the destination address with bitshifts. |
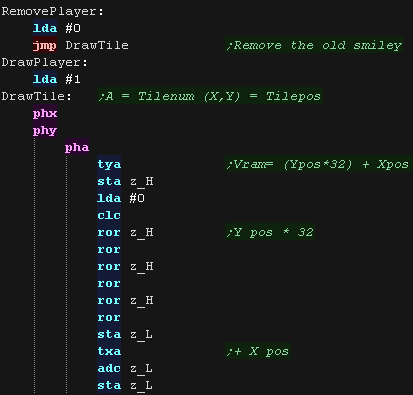 |
We now need to wait for VBLANK, as we cannot write to VRAM outside
of VBLANK. |
 |
Next we select the VRAM address width ports 2116/7, write the two
bytes to select the tile pattern of the character we want to show
with 2119/8
(The top byte is zero, the bottom is the pattern number). |
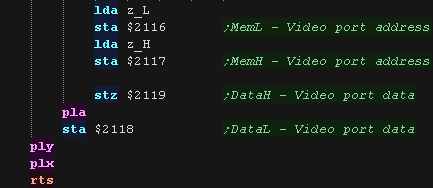 |
 |
Tiles are OK for simple
graphics and games - for more smooth moves we'd want to use hardware
sprites... But they're a bit more tricky, so make do with tiles to
get started!!! |
Moving our smiley!
During our main loop, the X and Y will be 8 bits only, We'll use
them as our smiley position.
|
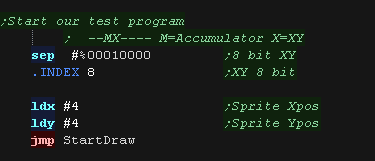 |
We have a 'ReadJoystick' routine that reads in all the directions
of the joypad.
We have to 'Strobe' the joypad to reset it. We write 1 then 0 to
port $4016 to do this.
We can then read in the buttons and directions one bit at a time
from $4016.
|
|
We use our ReadJoystick routine to get any movements, and move our
smiley as needed.
If our smiley is about to go off screen, we skip the movement.
We show the new sprite position after moving the sprite.
|
|
We then pause a moment to slow down the routine.
Because the SNES is very fast, we need a big loop!
We switch the X register to 16 bit for the delay, before switching
it back to 8 bit.
|
|
| |
Buy my Assembly programming book on Amazon in Print or Kindle!
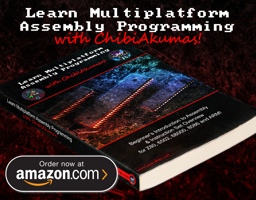
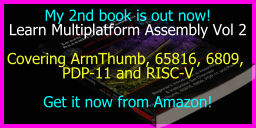
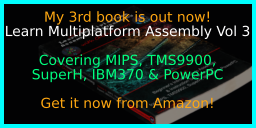
Available worldwide! Search 'ChibiAkumas' on your local Amazon website!
Click here for more info!
Buy my Assembly programming book on Amazon in Print or Kindle!
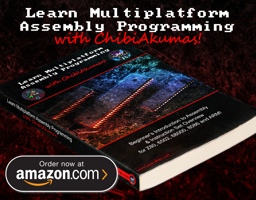
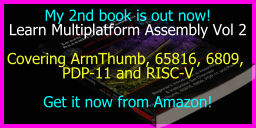
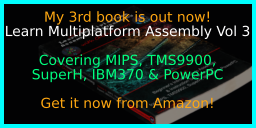
Available worldwide! Search 'ChibiAkumas' on your local Amazon website!
Click here for more info!
Buy my Assembly programming book on Amazon in Print or Kindle!
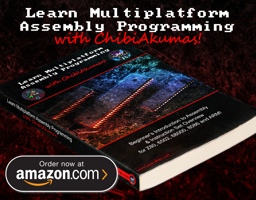
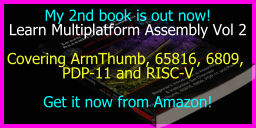
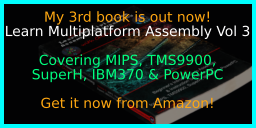
Available worldwide! Search 'ChibiAkumas' on your local Amazon website!
Click here for more info!
|