WLADX needs us to define the layout of our memory. We're defining
a simple single block of memory $20000 in size. |
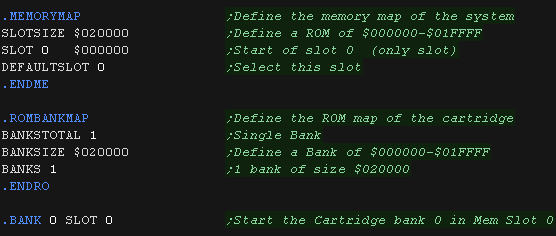 |
We define some direct page entries for our work.
Z_HL is made up of 3 bytes U, H and L for 24 bit addresses.
We also have a CursorX/Y for the next character position. |
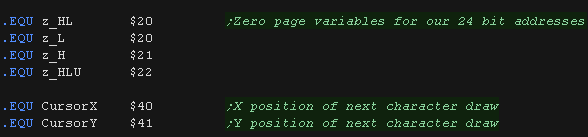 |
Our cartridge starts at address $8000.
We begin by stopping interrupts, enabling 65816 mode, and setting
the X/Y registers to 16 bit.
The Accumulator and memory functions are still 8 bit. |
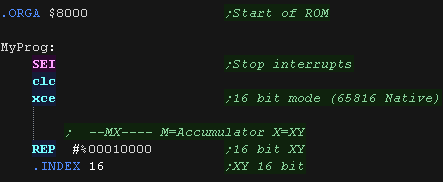 |
We need to set up our screen!
Unfortunately there's a lot of strange settings we need to set to do
this, but we've got basic values here to set up a screen with a
32x32 tilemap. |
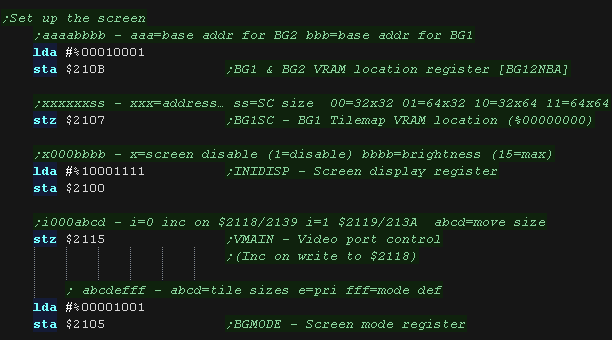 |
We need to set up the colors for our screen. The background is
color 0, the font is color 3.
|
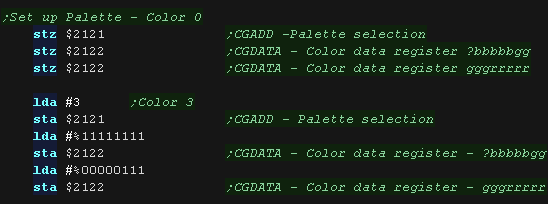 |
We need to set up a font. We've defined bytes for a 1 bit per
pixel font in the code.
|
|
We need to transfer our font into pattern RAM.
We load the address of our font into the direct page address z_HL,
and copy the bytes into pattern RAM.
Each line of pattern RAM takes 4 bytes.
We send the first two in one block (Bitplanes 0/1), and the matching
second two in the second block (Bitplanes 2/3).
We send one line of our font data twice in the first block, and zero
bytes in the second block.
This has the effect of setting our font as color 3.
|
|
We need to set the scroll position of the tilemap, and zero all
the tiles in the tilemap.
The tilemap is 32x32, so there are $400 entries, and each one takes
2 bytes.
|
|
Now all our data has been transferred, we can turn
on our screen.
|
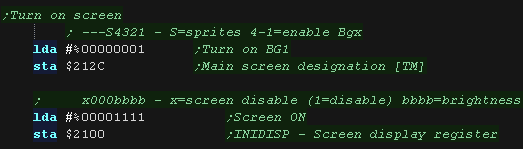 |
Our test program loads the address of the 'Hello World' string
into the Z_HL zero page entry, and calls the 'PrintString'
subroutine.
It then stops the processor with an infinite loop. |
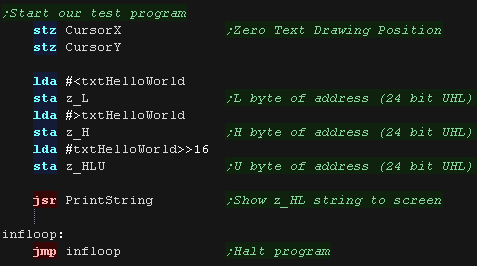 |
The 'PrintString' routine simply reads one byte at a time from the
z_HL direct page.
When we get to the 255 byte we return, otherwise we pass the
character to the 'PrintString' routine. |
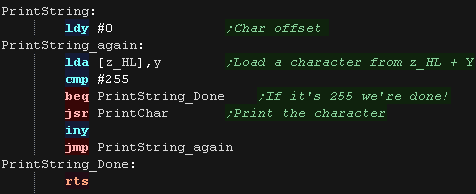 |
The PrintChar routine will print the character to the screen using
the tilemap.
First we need to convert ASCII to the pattern number.
We start by converting lowercase to uppercase, as we have no
lowercase in our font.
Next we subtract 64, as our first character is 'A', This converts to
the correct tile pattern number. |
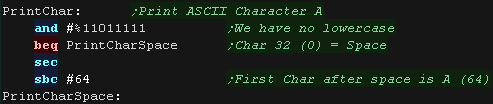 |
We now need to calculate the VRAM address.
The tilemap is at VRAM address $0000 onwards, and each line is 32
tiles, so the formula is 'VRAM = (Ypos*32) + Xpos'.
We calculate the destination address with bitshifts. |
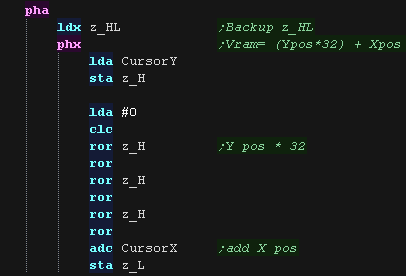 |
We now need to wait for VBLANK, as we cannot write to VRAM outside
of VBLANK. |
 |
Next we select the VRAM address, write the two bytes to select the
tile pattern of the character we want to show
(The top byte is zero, the bottom is the pattern number).
We then increase our CursorX position, and return. |
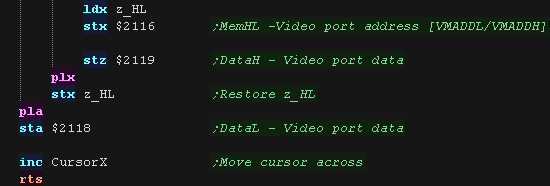 |
At the end of our cartridge we need to define a reset vector,
which points to the start of our cartridge. |
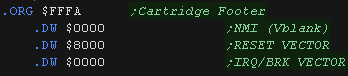 |