68000 Assembly programming for
the Sharp x68000 (x68k)
The X68000 is the Japanese exclusive
PC type computer... with hardware virtually identical to the Capcom
CPS hardware, it has great arcade ports, to the extent that many
arcade games were developed on it!
Released in 1987, it had hardware sprites and FM sound long before
the IBM PC, however it's DOS-like Human68k is easy for someone used
to the PC to pick up.
The X6800 is instantly recognizable by it's 'Twin Tower' (Manhattan)
design, unfortunately, its continuing popularity means it's
expensive to buy, and it's power supply is prone to failure... it
also needs a special keyboard and mouse - and cannot use PS2, which
adds to the cost of ownership... it can however use MSX joypads!
|
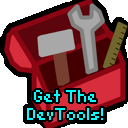 |
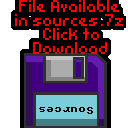 |
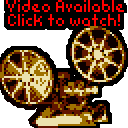 |
Lets take a look at the X68000 specs!
Specs:
|
x68000 base
model |
Cpu |
10mhz |
Ram |
1MB |
Vram |
1MB |
Resolution |
768x512... 512x512... 256,256 |
Bitmap planes |
4 @ 512x512 16 color |
Max Sprites |
128 sprites 16 color (16x16 px 32 per line) |
Sound chip |
FM (YM2151) + ADPCM |
|
|
ChibiAkumas Tutorials
Documentation
GamesX -
Great source of English x68000 info
X68000 technical data book
- It's in Japanese, but I've needed it many times in my programming, so what
can you do?
YM2151 details - Full
details on the X68000 FM sound Chip
Hello World for the
X68000
We can build Hello World with VASM,
or the official assembler.
If we build with VASM we should output in binary, and add our own
Xfile header so we can run the program
the Sharp/Hudson assembler and linker will output a complete xfile
for us! |
VASM version |
Sharp/Hudson x68
assembler |
pea mes
;Push message addres
dc.w $ff09
;show string
addq.l #4,SP ;skip over
pushed message
dc.w $FF00
;return
mes: dc.b 'Hello World',$0D,$0A,0 |
pea mes
.dc.w $ff09
addq.l #4,sp
.dc.w $ff00
mes: .dc.b 'hello',$0d,$0a,0 |
|
Setting Graphics Modes
Rather than using system calls, We can select a graphics mode by writing the
correct values to registers $e80000-$e8002e
Here are some sample values for a 16 color screen ... you will need to
change the values in the dark section if you want 256 colors.
|
High Resolution |
Low Resolution |
|
RegNum |
768x512 |
512x512 |
512x256 |
256x256 |
512x512 |
512x256 |
256x256 |
Register Purpose |
E80000 |
$89 |
$5B |
$5B |
$2B |
$4B |
$4B |
$25 |
R00 Horizontal total |
E80002 |
$0E |
$09 |
$09 |
$04 |
$03 |
$03 |
$01 |
R01 Horizontal synchronization end position
timing |
E80004 |
$1C |
$11 |
$11 |
$06 |
$04 |
$05 |
$00 |
R02 Horizontal display start position |
E80006 |
$7C |
$51 |
$51 |
$26 |
$45 |
$45 |
$20 |
R03 Horizontal display end position |
E80008 |
$237 |
$237 |
$237 |
$237 |
$103 |
$103 |
$103 |
R04 Vertical total |
E8000A |
$05 |
$05 |
$05 |
$05 |
$02 |
$02 |
$02 |
R05 Vertical synchronization end position
timing |
E8000C |
$28 |
$28 |
$28 |
$28 |
$10 |
$10 |
$10 |
R06 Vertical display start position |
E8000E |
$228 |
$228 |
$228 |
$228 |
$100 |
$100 |
$100 |
R07 Vertical display end position |
E80010 |
$1B |
$1B |
$1B |
$1B |
$2C |
$2C |
$24 |
R08 External synchronization horizontal
adjust: Horizontal position tuning |
E80028 |
$416
|
$415
|
$411 |
$410 |
$05 |
$01 |
$00 |
R20 Memory mode/Display mode control |
E82400 |
$04
|
$04 |
$04
|
$04 |
$00 |
$00 |
$00 |
R0 (Screen mode initialization) - Detail |
E82500 |
$2E4 |
$2E4 |
$2E4 |
$2E4 |
$2E4 |
$2E4 |
$2E4 |
R1 (Priority control) - Priority (Sprites
foreground) |
E82600 |
$DF
|
$DF |
$DF |
$DF |
$C1 |
$C1 |
$C1 |
R2 (Special priority/screen display) -
Screen On / Sprites On |
EB0808 |
$200 |
$200 |
$200 |
$200 |
$200 |
$200 |
$200 |
BG Control (Sprites Visible, slow writing) |
EB080A |
|
$FF |
$FF |
$FF |
$FF |
$FF |
$25 |
Sprite H Total |
EB080C |
|
$15 |
$15 |
$0A |
$09 |
$09 |
$04 |
Sprite H Disp |
EB080E |
|
$28 |
$28 |
$28 |
$10 |
$10 |
$10 |
Sprite V Disp |
EB0810 |
|
$15 |
$11 |
$10 |
$05 |
$01 |
$00 |
Sprite Res %---FVVHH |
Palette Definitions
Graphics mode palettes are defined by the registers from
$e82000... each takes 2 bytes, so color 0 is at $e82000, and color 1
is at $e82002... there are up to 256 depending on screen mode.
Each color is defined by 5 bits per channel in the format shown to
the right
Text palettes are in the same format from $e82200 - there are 16
Sprite palettes are in the same format from $e82200 - there are 240 |
F |
E |
D |
C |
B |
A |
9 |
8 |
|
7 |
6 |
5 |
4 |
3 |
2 |
1 |
0 |
G4 |
G3 |
G2 |
G1 |
G0 |
R4 |
R3 |
R2 |
|
R1 |
R0 |
B4 |
B3 |
B2 |
B1 |
B0 |
- |
|
Joypad Reading
$E9A001 reads player 1, $E9A003 reads player 2
$E9A005 selects which buttons we're reading in
|
Address |
Purpose |
Bits |
Notes |
$E9A001 |
Joystick
#
1 |
|
Data
from
Joy 1 |
$E9A003 |
Joystick
#
2 |
|
Data
from
Joy 2 |
$E9A005 |
Joystick
Control |
--MM---- |
00
Select
Normal / 11 alt keys |
|
Depending on the bits we write to
$E9A005, the data we get back from the two Joystick ports will
either return the basic joystick buttons, or the extra buttons of a
genesis joypad. |
Byte
written to
$E9A005 |
Purpose |
$E9A001
/ $E9A003
Data Bits |
%00000000 |
Basic
Buttons |
%-21-RLDU |
%00110000 |
Extra
Buttons |
%-S3-M654 |
|
FM Sound - YM2151 Chip
For full details of the YM2151 can be found in the YM2151
PDF
The FM sound chip has 8 channels....
Each channel's sound can be built up with 4 different 'slots'...
meaning there are a total of 32 slots... these slots are turned on
or off when the sound is triggered
Setting a register is easy, we write the register number to
$E90001 , then we write the 8
bit value to $E90003
For registers with 32 slots (eg $60 - volume) we can calculate the
address of a channels slot with the formula:
Address = RegisterBase + 8*ChannelSlot
+ Channel
So if RegisterBase=$60 , ChannelSlot=3
and Channel=7 then we get $60+24+7 |
Setting
a register on the X68000
move.b #$20,$E90001
move.b #%11000000,$E90003 |
YM2151 Registers
The YM2151 is controlled by 255 registers, that are summarized below:
Address |
7 |
6 |
5 |
4 |
3 |
2 |
1 |
0 |
Summary |
Bit
Meanings |
$01 |
T |
T |
T |
T |
T |
T |
T |
T |
Test |
T=Test |
$08 |
- |
S |
S |
S |
S |
C |
C |
C |
Key On (Play
Sound) |
C=Channel
S=Slot (C2 � M2 � C1 � M1) |
$0F |
E |
- |
- |
F |
F |
F |
F |
F |
Noise |
E=noise
enable F=Frequency
(Noise only on Chn7 Slot32)
|
$10 |
C |
C |
C |
C |
C |
C |
C |
C |
CLKA1 |
|
$11 |
- |
- |
- |
- |
- |
- |
C |
C |
CLKA2 |
|
$12 |
C |
C |
C |
C |
C |
C |
C |
C |
CLKB |
|
$14 |
C |
- |
F |
F |
I |
I |
L |
L |
|
C=CSM
F=F-Reset I=IRQEN L=LOAD |
$18 |
L |
L |
L |
L |
L |
L |
L |
L |
LFREQ |
|
$19 |
M |
M |
M |
M |
M |
M |
M |
M |
PMD/AMD |
|
$1B |
D |
C |
- |
- |
- |
- |
W |
W |
|
D=Disk
state C=CT
(4mhz/8mhz)
W=Waveform (0=Saw 1=Square,2=Tri, 3=Noise)
|
$20-$27 |
L |
R |
F |
F |
F |
C |
C |
C |
Chn0-7� |
F=Feedback,
C=Connection |
$28-$2F |
- |
O |
O |
O |
N |
N |
N |
N |
Chn0-7�
KeyCode |
O=Octave,
N=Note |
$30-$37 |
F |
F |
F |
F |
F |
F |
- |
- |
Chn0-7� Key
Fraction |
F=Fraction |
$38-$3F |
- |
P |
P |
P |
- |
- |
A |
A |
Chn0-7� PMS /
AMS |
P=PMS , A=AMS |
$40-$5F |
- |
D |
D |
D |
M |
M |
M |
M |
Slot1-32.
Decay/Mult |
D=Decay D1T,
M=Mult |
$60-$7F |
- |
V |
V |
V |
V |
V |
V |
V |
Slot1-32.
Volume |
V=Volume (TL)
(0=max)
|
$80-$9F |
K |
K |
- |
A |
A |
A |
A |
A |
Slot1-32.
Keyscale / Attack |
K=Keycale,
A=attack |
$A0-$BF |
A |
- |
- |
D |
D |
D |
D |
D |
Slot1-32. AMS
/ Decay |
A=AMS-EN,
D=Decay D1R |
$C0-$DF |
T |
T |
- |
D |
D |
D |
D |
D |
Slot1-32.
DeTune / Decay |
T=Detune DT2,
D=Decay D2R |
$E0-$FF |
D |
D |
D |
D |
R |
R |
R |
R |
Slot1-32.
Decay / Release |
D=Decay D1L,
R=Release Rate |
Not all values for NNNN in the Octave in $28-$2F have a different note,
the following are useful:
NNNN Value |
0
|
1
|
2
|
4
|
5
|
6
|
8
|
9 |
10
|
12
|
13
|
14
|
Note |
C# |
D |
D# |
E |
F |
F# |
G |
G# |
A |
A# |
B |
C |
Connection options for $20-$27 are shown below:
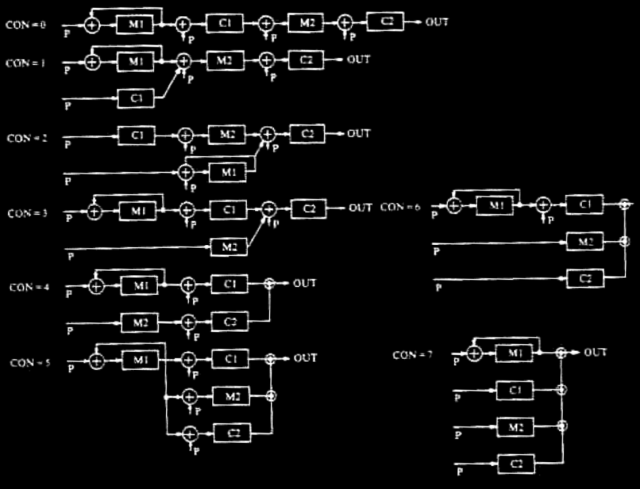
Vblank!
I had a lot of trouble finding documentation on how to wait for
Vblank, so I though I'd mark it clearly here!
Vblank can be detected by the MFP (MC68901)... By testing memory
address $e88001 - bit 4
The sample code I used for Grime68000 is shown to the right... |
waitVBlank:
move.w $e88000,d0
and.w #%00010000,d0
;Wait for
vblank to start
beq waitVBlank
waitVBlank2:
move.w $e88000,d0
and.w #%00010000,d0
;Wait for
Vblank to end
bne waitVBlank2
rts |
Hardware
Sprites - Initialization
We need to set up some registers to
get our sprites working - otherwise they just won't show!
We have to set up our layering correctly, so the sprites are in the
foreground, we do this by setting $E82500 to $2E4
We have to enable the sprite layer, we do this by setting $E82600 to
$C1
We also need to set the "Background Control"... we're going to make
the sprites visible, this slows down writing, but means we can
change the sprites while showing them... we do this by writing
$200 to $EB0808
We also need to set Registers $EB080A-$EB0810 to the values shown in
the 'Setting Graphics Modes' Table
|
move.w #%0000001000000000,$eB0808
;Disp/CPU 1=sprites on (slow writing)
move.w
#%0000001011100100,$e82500
;R1 (Priority control) - Priority
move.w #%0000000011000001,$e82600
;R2 (Special priority/screen display) - Screen
On - sprites on
move.w #%0000001000000000,$eB0808
;Disp/CPU 1=sprites on (slow writing)
move.w
#$25,$EB080A
; Sprite H Total
move.w
#$04,$EB080C
; Sprite H Disp
move.w
#$10,$EB080E
; Sprite V Disp
move.w
#$00,$EB0810
; Sprite Res %---FVVHH |
Sprite
Settings
Each Sprite has 4 Words defining the settings of the
sprite... these start at $EB0000 for sprite 0....
$EB0008 for sprite 1, through to $EB03F8 for sprite 127
Address |
F |
E |
D |
C |
B |
A |
9 |
8 |
|
7 |
6 |
5 |
4 |
3 |
2 |
1 |
0 |
|
$EB0000 |
- |
- |
- |
- |
- |
- |
X |
X |
|
X |
X |
X |
X |
X |
X |
X |
X |
X=Xpos (16 is far left)
|
$EB0002 |
- |
- |
- |
- |
- |
- |
Y |
Y |
|
Y |
Y |
Y |
Y |
Y |
Y |
Y |
Y |
Y=Ypos (16 is top)
|
$EB0004 |
V |
H |
- |
- |
C |
C |
C |
C |
|
S |
S |
S |
S |
S |
S |
S |
S |
V=Vflip, H=Hflip, C=color,
S=sprite |
$EB0006 |
- |
- |
- |
- |
- |
- |
- |
- |
|
- |
- |
- |
- |
- |
- |
P |
P |
P=Priority (00=off... 01=back... 11=front) |
Sprite Bitmap Data Settings
Sprite bitmap data appears from address $EB8000
onwards, each sprite is 128 bytes...
Sprites are split into four 8x8 chunks, these are stored in 4 different
memory addresses to make up the 16x16 sprite,
The byte data for these sprites uses 1 nibble for each pixel, and selects a
color from the chosen palette for that pixel
Sprite Pixel Data:
Address |
F |
E |
D |
C |
B |
A |
9 |
8 |
7 |
6 |
5 |
4 |
3 |
2 |
1 |
0 |
EB8000 |
Color |
Color |
Color |
Color |
EB8002 |
Color |
Color |
Color |
Color |
� |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
EBBFFE |
Color |
Color |
Color |
Color |
8x8 Chunk layout:
|
16 pixels |
16 pixels |
TopLeft
1
|
TopRight
3
|
BottomLeft
2
|
BottomRight
4
|
|
8x8 chunks position in ram:
SpriteNum |
8x8
chunk |
Address |
0 |
TopLeft |
$EB8000 |
BottomLeft |
$EB8020 |
TopRight |
$EB8040 |
BottomRight |
$EB8060 |
1 |
TopLeft |
$EB8080 |
BottomLeft |
$EB80A0 |
TopRight |
$EB80C0 |
BottomRight |
$EB80E0 |
2 |
TopLeft |
$EB8100 |
|
� |
|
127 |
TopLeft |
$EBBF80 |
BottomLeft |
$EBBFA0 |
TopRight |
$EBBFC0 |
BottomRight |
$EBBFE0 |
|
|
X68000 3D Shutter
Glasses
The X68000 supports 3D shutter glasses via the external port...
generic shutter glasses can be connected via a converter.
Unfortunately they don't seem to be supported by any available
emulator!
The shutter glasses are controlled by bit 0/1 of port $E8E003...
Writing 0 opens a lens, writing 1 closes a lens. |
address |
Bytes |
Function |
Bits |
$E8E003 |
1
Byte (RW)
|
3D
Shutter Glasses |
%----T-LR |
|
Mouse
The X68000 Mouse can be controlled via Trap #15 - the option is selected
with the value in D0.
The operating system can show a cursor and even create a limited range
(handy for games like DUNE 2), but the mouse cursor can be read with no
cursor visible, and 'movement amounts' can be measured with $74 (handy for
games like DOOM)
Trap #15
D0 |
Name |
Function |
Params |
Returns |
Notes |
$70 |
_MS_INIT |
Mouse Init |
|
|
|
$71 |
_MS_CURON |
Cursor On |
|
|
|
$72 |
_MS_CUROF |
Cursor Off |
|
|
|
$73 |
_MS_STAT |
Get Cursor Status |
|
D0=status |
|
$74 |
_MS_GETDT |
Get Buttons + Move amount |
|
D0=$XXYYLLRR |
|
$75 |
_MS_CURGT
|
Get Cursor Pos |
|
D0=$XXXXYYYY |
|
$76 |
_MS_CURST |
Set Cursor Pos |
D1=$XXXXYYYY |
D0=Success? |
|
$77 |
_MS_LIMIT |
Set Range Limits (X,Y)-(x,y) |
D1=$XXXXYYYY D2=$xxxxyyyy |
|
|
$78 |
_MS_OFFTM |
Check time until mouse button was released |
D1=Button (0/1=L/R) D2=Wait Time |
D0=Drag/Wait |
|
$79 |
_MS_ONTM |
Time until mouse button pressed |
D1=Button (0/1=L/R) D2=Wait Time |
D0=Drag/Wait |
|
$7A |
_MS_PATST |
Set Cursor Graphic |
D1=PatternNum A1=Graphic Address |
|
18 words�
1W: center pos X
1W: centercPos Y
16W: Shadow graphic
16W: Cusor Graphic |
$7B |
_MS_SEL |
Select Cursor Graphic |
D1=PatternNum |
|
|
$7C |
_MS_SEL2 |
Select Animated Cursor |
A1=Graphic Address |
|
Up to 6 words�
Each is a cursor number -1=End |
$7D |
_SKEY_MOD |
Show/Hide Soft Keyboard
|
D1=0/1/2/-1 (Off/On/Check/Auto) D2=$XXXXYYYY
|
D0=State
|
|
Bitmap Layer addresses
(16 color mode)
Here are the addresses you need to write to for each layer!... Don't
forget to turn the layers on with $e82600, and set the priority with
$e82500!!!
Layer
|
VRAM base
|
Scroll-X
|
Scroll-Y
|
0
|
$c00000 |
$e80018 |
$e8001a |
1
|
$c80000 |
$e8001c |
$e8001e |
2
|
$d00000 |
$e80020 |
$e80022 |
3
|
$d80000 |
$e80024 |
$e80026 |
Text Layer addresses
(16 color)
Each pixel can be color 0-15... 4 bits per pixel... This data is split
into bitplanes, meaning all the 'bit 0's of the pixels are stored
together, as are bit 1's , 2's and 3's
Bit 0's are at $E00000, Bit 1's are at $E20000 and so on.
Address |
Bitplane |
$E00000
|
0 |
$E20000 |
1 |
$E40000 |
2 |
$E60000 |
3 |
Palette: $E82200-$E8221E
(%GGGGGRRRRRBBBBBT T=Transparent) (Same as Sprite Palette 0)
Scroll-X: $E80014
Scroll-Y: $E80016
Don't forget to turn the layers on with $E82600, and set
the priority with $E82500!!!
Memory Map and
Hardware Registers
address |
vector |
|
Function |
|
$000000 |
$00 |
|
SSP
after
reset |
|
$000004 |
$01 |
|
PC
after
reset |
|
$000008 |
$02 |
|
Bus
error |
|
$00000c |
$03 |
|
Address
error |
|
$000010 |
$04 |
|
Unknown
instruction |
|
$000014 |
$05 |
|
Division
by
0 |
|
$000018 |
$06 |
|
CHK
instruction |
|
$00001c |
$07 |
|
TRAPV
instruction,
FTRAPcc instruction |
|
$000020 |
$08 |
|
Privilege
violation |
|
$000024 |
$09 |
|
Trace
exception |
|
$000028 |
$0a |
|
Unsupported
instruction
line 1010 emulator (SX call) |
|
$00002c |
$0b |
|
?
line 1111 emulator (DOS call, floating point operation) |
|
$000030 |
$0c |
|
Unused |
|
$000034 |
$0d |
|
FPU
????????????????? |
|
$000038 |
$0e |
|
?
????????????? |
|
$000034 |
$0d |
|
FPU
Protocol
violation exception handling |
|
$000038 |
$0e
'' |
|
Formatting
error
exception handling |
|
$00003c |
$0f |
|
Uninitialized
Interrupt |
|
$000040 |
$10 |
|
Unused |
|
$000044 |
$11 |
|
? |
|
$000048 |
$12 |
|
? |
|
$00004c |
$13 |
|
? |
|
$000050 |
$14 |
|
? |
|
$000054 |
$15 |
|
? |
|
$000058 |
$16 |
|
? |
|
$00005c |
$17 |
|
? |
|
$000060 |
$18 |
|
Spurious
Interrupt |
|
$000064 |
$19 |
|
Level
1
Interrupt (auto vector) |
|
$000068 |
$1a |
|
? |
|
$00006c |
$1b |
|
? |
|
$000070 |
$1c |
|
? |
|
$000074 |
$1d |
|
? |
|
$000078 |
$1e |
|
? |
|
$00007c |
$1f |
|
? |
|
$000080 |
$20 |
|
trap
#0 |
|
$000084 |
$21 |
|
? #1 |
|
$000088 |
$22 |
|
? #2 |
|
$00008c |
$23 |
|
? #3 |
|
$000090 |
$24 |
|
? #4 |
|
$000094 |
$25 |
|
? #5 |
|
$000098 |
$26 |
|
? #6 |
|
$00009c |
$27 |
|
? #7 |
|
$0000a0 |
$28 |
|
? #8
(reserved for system) |
|
$0000a4 |
$29 |
|
? #9
(OS debugger) |
|
$0000a8 |
$2a |
|
? #10
(reset & power off) |
|
$0000ac |
$2b |
|
? #11
(BREAK key) |
|
$0000b0 |
$2c |
|
? #12
(COPY key) |
|
$0000b4 |
$2d |
|
? #13
(CTRL+C) |
|
$0000b8 |
$2e |
|
? #14
(error processing) |
|
$0000bc |
$2f |
|
? #15
(IOCS call) |
|
$0000c0 |
$30 |
|
FPU
BSUN |
|
$0000c0 |
$30 |
|
FPU
BSUN |
|
$0000c4 |
$31 |
|
?
INEX1,INEX2 |
|
$0000c8 |
$32 |
|
? DZ |
|
$0000cc |
$33 |
|
?
UNFL |
|
$0000d0 |
$34 |
|
?
OPERR |
|
$0000d4 |
$35 |
|
?
OVFL |
|
$0000d8 |
$36 |
|
?
SNAN |
|
$0000dc |
$37 |
|
??? |
|
$0000dc |
$37
|
|
Unused |
|
$0000e0 |
$38 |
|
MMU |
|
$0000e4 |
$39 |
|
? |
|
$0000e8 |
$3a |
|
? |
|
$0000ec |
$3b |
|
Unused |
|
$0000fc |
$3f |
|
Unused |
|
$000100 |
$40 |
|
MFP
RTC Alarm/1Hz |
|
$000104 |
$41 |
|
MFP
External power OFF |
|
$000118 |
$42 |
|
MFP
Front switch OFF |
|
$00010c |
$43 |
|
MFP
FM Audio source |
|
$000110 |
$44 |
|
MFP
Timer-D (Used with BG processing) |
|
$000114 |
$45 |
|
MFP
Timer-C (Mouse/cursor/FDD control, etc.) |
|
$000118 |
$46 |
|
MFP
V-DISP |
|
$00011c |
$47 |
|
MFP
RTC Clock |
|
$000120 |
$48 |
|
MFP
Timer-B |
|
$000124 |
$49 |
|
MFP
Key serial output error |
|
$000128 |
$4a |
|
MFP
Key serial output empty |
|
$00012c |
$4b |
|
MFP
Key serial input error |
|
$000130 |
$4c |
|
MFP
Key serial input |
|
$000134 |
$4d |
|
MFP
Timer-A |
|
$000138 |
$4e |
|
MFP
CRTC*IRQ |
|
$00013c |
$4f |
|
MFP
H-SYNC |
|
$000140 |
$50 |
|
SCC(B)
Transmission buffer empty |
|
$000144 |
$51 |
|
SCC(B)
'' |
|
$000148 |
$52 |
|
SCC(B)
External/status changes |
|
$00014c |
$53 |
|
SCC(B)
'' |
|
$000150 |
$54 |
|
SCC(B)
Incoming character validity (Mouse 1 byte input) |
|
$000154 |
$55 |
|
SCC(B)
'' |
|
$000158 |
$56 |
|
SCC(B)
Special Rx condition |
|
$00015c |
$57 |
|
SCC(B)
'' |
|
$000160 |
$58 |
|
SCC(A)
Transmission buffer empty |
|
$000164 |
$59 |
|
SCC(A)
'' |
|
$000168 |
$5a |
|
SCC(A)
External status changes |
|
$00016c |
$5b |
|
SCC(A)
'' |
|
$000170 |
$5c |
|
SCC(A)
Incoming character validity (RS-232C 1 byte input) |
|
$000174 |
$5d |
|
SCC(A)
'' |
|
$000178 |
$5e |
|
SCC(A)
Special Rx Condition |
|
$00017c |
$5f |
|
SCC(A)
'' |
|
$000180 |
$60 |
|
I/O
FDC status interruption |
|
$000184 |
$61 |
|
I/O
FDC insertion/discharge interruption |
|
$000188 |
$62 |
|
I/O
HDC status interruption |
|
$00018c |
$63 |
|
I/O
Printer ready interruption |
|
$000190 |
$64 |
|
DMAC
#0 End (FDD) |
|
$000194 |
$65 |
|
DMAC
#0 Error ('') |
|
$000198 |
$66 |
|
DMAC
#1 End (SASI) |
|
$00019c |
$67 |
|
DMAC
#1 Error ('') |
|
$0001a0 |
$68 |
|
DMAC
#2 End (IOCS _DMAMOVE,_DMAMOV_A,_DMAMOV_L) |
|
$0001a4 |
$69 |
|
DMAC
#2 Error ('') |
|
$0001a8 |
$6a |
|
DMAC
#3 End (ADPCM) |
|
$0001ac |
$6b |
|
DMAC
#3 Error ('') |
|
$000200 |
$6c |
|
SPC
SCSI interruption (Internal SCSI) |
|
$000204 |
$6d |
|
Unused |
|
$0003d4 |
$f5 |
|
Unused |
|
$0003d8 |
$f6 |
|
SPC
SCSI interruption (SCSI board) |
|
$0003dc |
$f7 |
|
Unused |
|
$0003fc |
$ff |
|
Unused |
|
0x000000 |
|
|
RAM
area |
|
$c00000 |
|
|
Graphics
Vram
� Page 0 |
|
$c80000 |
|
|
Graphics
Vram
� Page 1 (256/16 color only) |
|
$d00000 |
|
|
Graphics
Vram
� Page 2 (16 color only)
|
|
$d80000 |
|
|
Graphics
Vram
� Page 3 (16 color only) |
|
$e00000 |
|
|
Text
Vram
� Bitplane 0 |
|
$e20000 |
|
|
Text
Vram
� Bitplane 1
|
|
$e40000 |
|
|
Text
Vram
� Bitplane 2
|
|
$e60000 |
|
|
Text
Vram
� Bitplane 3
|
|
$e80000 |
1.w |
|
R00
Horizontal
total |
|
$e80002 |
1.w |
|
R01
Horizontal
synchronization end position timing |
|
$e80004 |
1.w |
|
R02
Horizontal
display start position |
|
$e80006 |
1.w |
|
R03
Horizontal
display end position |
|
$e80008 |
1.w |
|
R04
Vertical
total |
|
$e8000a |
1.w |
|
R05
Vertical
synchronization end position timing |
|
$e8000c |
1.w |
|
R06
Vertical
display start position |
|
$e8000e |
1.w |
|
R07
Vertical
display end position |
|
$e80010 |
1.w |
|
R08
External
synchronization horizontal adjust: Horizontal position tuning |
|
$e80012 |
1.w |
|
R09
Raster
number: Used for raster interruption |
|
$e80014 |
1.w |
|
R10
Text
Screen X coordinate |
|
$e80016 |
1.w |
|
R11
Text
Screen Y coordinate |
|
$e80018 |
1.w |
|
R12
Graphics
screen Scroll X0 |
|
$e8001a |
1.w |
|
R13
Graphics
screen Scroll Y0 |
|
$e8001c |
1.w |
|
R14
Graphics
screen Scroll X1 |
|
$e8001e |
1.w |
|
R15
Graphics
screen Scroll Y1 |
|
$e80020 |
1.w |
|
R16
Graphics
screen Scroll X2 |
|
$e80022 |
1.w |
|
R17
Graphics
screen Scroll Y2 |
|
$e80024 |
1.w |
|
R18
Graphics
screen Scroll X3 |
|
$e80026 |
1.w |
|
R19
Graphics
screen Scroll Y3 |
|
$e80028 |
1.w |
|
R20
Memory
mode/Display mode control |
|
$e8002a |
1.w |
|
R21
Simultaneous
access/Raster copy/Quick clear plane select |
|
$e8002c |
1.w |
|
R22
Raster
copy action: Raster number |
|
$e8002e |
1.w |
|
R23
Text
screen access mask pattern |
|
$e80481 |
1.b |
|
Active
Image
capture/Quick clear/Raster copy control |
|
$e82000 |
256.w |
|
Graphics
palette |
|
$e82200 |
16.w |
|
Text
palette
(Palette block 0) |
|
$e82220 |
240.w |
|
Sprite
palette
('' 1-15) |
|
$e82400 |
1.w |
|
R0
(Screen
mode initialization) |
|
$e82500 |
1.w |
|
R1
(Priority
control) |
|
$e82600 |
1.w |
|
R2
(Special
priority/screen display) - Layers On/Off
|
|
$e84000 |
|
|
DMAC
(HD63450) |
|
$e86000 |
|
|
Memory
controller
privileged access settings (OHM/ASA) |
|
$e88000 |
|
|
MFP
(MC68901) |
|
$e8a000 |
|
|
RTC
(RP5C15) |
|
$e8c000 |
|
|
Printer
port |
|
$e8e001 |
|
|
#1
Contrast |
|
$e8e003 |
|
|
#2
Display/3D
Shutter Glasses (Bit 0=Right Eye / Bit 1 = Left Eye)
|
|
$e8e005 |
|
|
#3
Color
image unit (bit 4-0) |
|
$e8e007 |
|
|
#4
Keyboard/NMI/dot
clock |
|
$e8e009 |
|
|
#5
ROM/DRAM
Wait |
|
$e8e00b |
|
|
#6
MPU
Classification/Operation clock |
|
$e8e00d |
|
|
#7
SRAM
Write |
|
$e8e00f |
|
|
#8
Unit
power OFF |
|
$E90001 |
|
|
FM Synthesizer (YM2151) -
Register Address Write port |
|
$E90003 |
|
|
FM
Synthesizer
(YM2151) - Data R/W port |
|
$E92000 |
|
|
ADPCM
(MSM6258V) |
|
$E94000 |
|
|
Floppy
disk
controller (FDC) (uPD72065) |
|
$E94005 |
|
|
Floppy
drive
monitor (IOSC) |
|
$E96000 |
|
|
SASI |
|
$E98000 |
|
|
ESCC
(Z8530) |
|
$E9A000 |
|
|
PPI
(82C55) |
|
$E9C000 |
|
|
I/O
selector
(IOSC) |
|
$E9E000 |
|
|
I/O
expansion
area (Sharp reserved) |
|
$EB0000 |
|
|
Sprite
register
(CYNTHIA) |
|
$EB8000 |
|
|
Sprite
VRAM |
|
$EC0000 |
|
|
I/O
expansion
area (User) |
|
$ed0072 |
2.b |
|
SX-Window
environment
flag (While in use with "SX") |
|
$ed0074 |
1.b |
|
Standard
double-click
time / 10 |
|
$ed0075 |
1.b |
|
Mouse
speed
/ 2 |
|
$ed0076 |
1.b |
|
Text
palette
hue (HSV) |
|
$ed0077 |
1.b |
|
|
|
$ed0078 |
1.b |
|
Brightness
palette
0-3 5bit??? |
|
$ed007b |
1.b |
|
Printer
drive
(PRTD) ID |
|
$ed007c |
1.b |
|
SRAM
info
version#, screen status storage, start screen storage |
|
$ed007d |
1.b |
|
Desktop
background
(PICT) ID |
|
$ed007e |
1.b |
|
Screen
mode |
|
$ed007f |
17.b |
|
Reserved
for
system use (X68030) |
|
$ed0090 |
1.b |
|
Standard
cache
status (bit=0: off 1:on) |
|
$ed0091 |
1.b |
|
OPM
music
during startup (0: OFF -1: ON) |
|
$ed0092 |
1.b |
|
10MHz
Proper
wait value |
|
$ed0093 |
1.b |
|
16MHz
'' |
|
$ed0094 |
108.b |
|
Reserved
for
system use |
|
$ed0100 |
768.b |
|
Head
SRAM
program address |
|
$ed0400 |
15KB |
|
Head
SRAMDISK
address |
|
$ed3fff |
|
|
End
of
SRAM |
|
$ed4000 |
|
|
Backup
(64KB) |
|
$ee0000 |
|
|
Unused
(128KB) |
|
$f00000 |
|
|
CGROM(768KB) |
|
$fc0000 |
|
|
SCSI
IOCS
/ IPL(8KB) |
|
$fe0000 |
|
|
ROM
Debugger |
|
$ff0000 |
|
|
IPL
/
ROM IOCS |
|
Dos Calls
You can use Dos calls to do basic tasks, they are performed with dc.w $FFxx
... where xx is the command number:
Code |
Shortname |
Meaning |
Example
|
Example function |
$FF00 |
_EXIT |
Program end |
dc.w $FF00 |
|
$FF01 |
_GETCHAR |
Get keyboard input (with echo) |
|
|
$FF02 |
_PUTCHAR |
Put character |
move.w #'A',-(a7)
dc.w $FF02
|
Show character 'A' to the screen
|
$FF03 |
_COMINP |
RS-232C 1 byte input |
|
|
$FF04 |
_COMOUT |
RS-232C 1 byte output |
|
|
$FF05 |
_PRNOUT |
Printer 1 character output |
|
|
$FF06 |
_INPOUT |
Character I/O |
|
|
$FF07 |
_INKEY |
Get one character from the
keyboard (no break check) |
|
|
$FF08 |
_GETC |
Get one character from the
keyboard (with break check) |
|
|
$FF09 |
_PRINT |
Print string |
pea mes
dc.w $ff09
mes:dc.b 'Hello World',$0D,$0A,0 |
Print 0 terminated 'mes' to screen |
$FF0A |
_GETS |
Get character string (with
break check) |
|
|
$FF0B |
_KEYSNS |
Key input state check |
|
|
$FF0C |
_KFLUSH |
Keyboard input after buffer
flush |
|
|
$FF0D |
_FFLUSH |
Disk reset |
|
|
$FF0E |
_CHGDRV |
Current drive setting |
|
|
$FF0F |
_DRVCTRL |
Drive status check/setting |
|
|
$FF10 |
_CONSNS |
Screen output check |
|
|
$FF11 |
_PRNSNS |
Printer output check |
|
|
$FF12 |
_CINSNS |
RS-232C input check |
|
|
$FF13 |
_COUTSNS |
RS-232C output check |
|
|
$FF17 |
_FATCHK |
File concatenation state check |
|
|
$FF18 |
_HENDSP |
Kanji conversion control |
|
|
$FF19 |
_CURDRV |
Get current drive |
|
|
$FF1A |
_GETSS |
Get character string (no break
check) |
|
|
$FF1B |
_FGETC |
Get character from file |
|
|
$FF1C |
_FGETS |
Get string from file |
|
|
$FF1D |
_FPUTC |
Write one character to file |
|
|
$FF1E |
_FPUTS |
Write string to file |
|
|
$FF1F |
_ALLCLOSE |
Close all files |
|
|
$FF20 |
_SUPER |
Supervisor/user mode setting |
clr.l d0
;0=Enable Supervisor mode
move.l d0,-(sp)
dc.w $FF20
;Switch Mode
|
0=Super Mode
!=0 User mode with new SP
|
$FF21 |
_FNCKEY |
Get/set redefinable key |
|
|
$FF22 |
_KNJCTRL |
Kana-to-kanji conversion |
|
|
$FF23 |
_CONCTRL |
Console control/direct output |
move.w #1,d2
move.w d2,-(sp)
move.w #2,d2
move.w d2,-(sp)
dc.w $ff23 |
Set console color to #1 |
|
|
|
move.w #6,d2
;Y
move.w d2,-(sp)
move.w #5,d2
;X
move.w d2,-(sp)
move.w #3,d2
move.w d2,-(sp)
dc.w $ff23 |
Set Cursor XY pos to 5,6 |
|
|
|
move.w #$12,-(a7)
dc.w $FF23 |
Cursor Off |
|
|
|
move.w #$03,-(a7)
move.w #$0E,-(a7)
dc.w $FF23 |
Function key display off |
$FF24 |
_KEYCTRL |
Console state check/direct
input |
|
|
$FF25 |
_INTVCS |
Set vector processing address |
|
|
$FF26 |
_PSPSET |
Create process management
pointer |
|
|
$FF27 |
_GETTIM2 |
Get time (longword) |
|
|
$FF28 |
_SETTIM2 |
Set time (longword) |
|
|
$FF29 |
_NAMESTS |
Filename expansion |
|
|
$FF2A |
_GETDATE |
Get date |
|
|
$FF2B |
_SETDATE |
Set date |
|
|
$FF2C |
_GETTIME |
Get time |
|
|
$FF2D |
_SETTIME |
Set time |
|
|
$FF2E |
_VERIFY |
Set verify flag |
|
|
$FF2F |
_DUP0 |
Force file handle copy |
|
|
$FF30 |
_VERNUM |
Get OS version |
|
|
$FF31 |
_KEEPPR |
Terminate and stay resident |
|
|
$FF32 |
_GETDPB |
Get drive parameter block |
|
|
$FF33 |
_BREAKCK |
Set break check |
|
|
$FF34 |
_DRVXCHG |
Replace drive |
|
|
$FF35 |
_INTVCG |
Get vector processing address |
|
|
$FF36 |
_DSKFRE |
Get disk space remaining |
|
|
$FF37 |
_NAMECK |
Filename expansion |
|
|
$FF39 |
_MKDIR |
Create subdirectory |
|
|
$FF3A |
_RMDIR |
Remove subdirectory |
|
|
$FF3B |
_CHDIR |
Change current directory |
|
|
$FF3C |
_CREATE |
Create file |
|
|
$FF3D |
_OPEN |
Open file |
|
|
$FF3E |
_CLOSE |
Close file |
|
|
$FF3F |
_READ |
Read file |
|
|
$FF40 |
_WRITE |
Write file |
|
|
$FF41 |
_DELETE |
Delete file |
|
|
$FF42 |
_SEEK |
Seek file |
|
|
$FF43 |
_CHMOD |
Set/get file modes |
|
|
$FF44 |
_IOCTRL |
Device driver ioctrl direct I/O |
|
|
$FF45 |
_DUP |
Copy file handle |
|
|
$FF46 |
_DUP2 |
Force copy file handle |
|
|
$FF47 |
_CURDIR |
Get current directory |
|
|
$FF48 |
_MALLOC |
Allocate memory |
|
|
$FF49 |
_MFREE |
Free memory |
|
|
$FF4A |
_SETBLOCK |
Change memory block |
|
|
$FF4B |
_EXEC |
Load/execute program |
|
|
$FF4C |
_EXIT2 |
Exit with return code |
move.w #1,-(sp)
dc.w $FF4C |
|
$FF4D |
_WAIT |
Get process end return code |
|
|
$FF4E |
_FILES |
Search files |
|
|
$FF4F |
_NFILES |
Search next files |
|
|
$FF80 |
_SETPDB |
Change process information |
|
|
$FF81 |
_GETPDB |
Get process information |
|
|
$FF82 |
_SETENV |
Set environment variable |
|
|
$FF83 |
_GETENV |
Get environment variable |
|
|
$FF84 |
_VERIFYG |
Get verify flag |
|
|
$FF85 |
_COMMON |
COMMON area control |
|
|
$FF86 |
_RENAME |
Rename/move file |
|
|
$FF87 |
_FILEDATE |
Get/set file date |
|
|
$FF88 |
_MALLOC2 |
Alloc memory |
|
|
$FF8A |
_MAKETMP |
Create temporary file |
|
|
$FF8B |
_NEWFILE |
Create new file |
|
|
$FF8C |
_LOCK |
Lock file |
|
|
$FF8F |
_ASSIGN |
Get/set/cancel virtual
drive/directory assignment |
|
|
$FFAA |
FFLUSH |
Set FFLUSH mode (undocumented) |
|
|
$FFAB |
_OS_PATCH |
Hook OS internal function
(undocumented) |
|
|
$FFAC |
_GETFCB |
Get FCB pointer (undocumented) |
|
|
$FFAD |
_S_MALLOC |
Alloc memory using main memory
management |
|
|
$FFAE |
_S_MFREE |
Free memory using main memory
management |
|
|
$FFAF |
_S_PROCESS |
Sub memory management setting |
|
|
$FFF0 |
_EXITVC |
(program end execution address) |
|
|
$FFF1 |
_CTRLVC |
(CTRL+C execution address at
abort) |
|
|
$FFF2 |
_ERRJVC |
(Error abort execution address) |
|
|
$FFF3 |
_DISKRED |
Block device direct input |
|
|
$FFF4 |
_DISKWRT |
Block device direct output |
|
|
$FFF5 |
_INDOSFLG |
Get OS work pointer |
|
|
$FFF6 |
_SUPER_JSR |
Supervisor subroutine call |
|
|
$FFF7 |
_BUS_ERR |
Check for bus error |
|
|
$FFF8 |
_OPEN_PR |
Register background task |
|
|
$FFF9 |
_KILL_PR |
Remove background task |
|
|
$FFFA |
_GET_PR |
Get thread management
information |
|
|
$FFFB |
_SUSPEND_PR |
Force thread to sleep |
|
|
$FFFC |
_SLEEP_PR |
Sleep thread |
|
|
$FFFD |
_SEND_PR |
Transmit thread command/data |
|
|
$FFFE |
_TIME_PR |
Get timer counter value |
|
|
$FFFF |
_CHANGE_PR |
Yield execution time |
|
|
Human68k
Human68k
is generally DOS like, however while it is based around 8.3 filenames, the
files can be 18.3 - but please note, the extra 10 letters are not 'really
counted' the first 8 must make the filename unique!
Human68k also supports lowercase files... but your disks will no longer be
compatible with MS-DOS
Most commands like CD, DIR and EXIT work the same as normal DOS, but many do
not, and some work differently ... here are a few commands beyond the basics
you may want to remember...
Command |
Meaning |
CUSTOM |
Reconfigure config.sys and autoexec.bat |
DISKCOPY A: B: |
Copy Disk A to B |
DUMP file.name |
Dump file.name as Hex/Ascii |
FORMAT B: |
erase Disk B |
SWITCH |
change bios settings (including installed memory) |
TYPE file.txt |
Type the contents of file.txt to the command line |
ED file.txt |
Edit file.txxt (see above section for usage) |
Interrupts and Vblank
Vblank and many other interrupts are generated by the MFT.
The interrupts can be enabled with $E88007/9, however they are also
'masked' with $E88013/5 (a bit 1 in both enables an interrupt!)
Address |
Function |
Bits |
Details |
$e88001 |
MFP:GPIP Data |
HI-VFPSA |
A=rtc Alarm
S=Slot �expwon� command P=power switch F=Fm irq V=Vblank I=video
Irq H=hblank |
$e88003 |
MFP:Active
Edge Reg |
HI-VFPSA |
A=rtc Alarm
S=Slot �expwon� command P=power switch F=Fm irq V=Vblank I=video
Irq H=hblank |
$e88005 |
MFP:GPIP port |
IIIIIIII |
IO Select |
$e88007 |
MFP:Interrupt
control A |
76AFRETB |
7=Gpip7
6=Gpip6 A=Timer A F=recieve buffer Full R=Recieve error E=send
buffer Empty T=transmit error B=timer B |
$e88009 |
MFP:Interrupt
control B |
54CD3210 |
5=gpip 5
4=gpip4 C=timer C D=timer D 3=gpip3 2=gpip2 1=gpip1 0=gpip 0 |
$e8800B |
MFP:Interrupt
Pending A |
76AFRETB |
7=Gpip7
6=Gpip6 A=Timer A F=recieve buffer Full R=Recieve error E=send
buffer Empty T=transmit error B=timer B |
$e8800D |
MFP:Interrupt
Pending B |
54CD3210 |
5=gpip 5
4=gpip4 C=timer C D=timer D 3=gpip3 2=gpip2 1=gpip1 0=gpip 0 |
$e8800F |
MFP:Interrupt
In service A |
76AFRETB |
7=Gpip7
6=Gpip6 A=Timer A F=recieve buffer Full R=Recieve error E=send
buffer Empty T=transmit error B=timer B |
$e88011 |
MFP:Interrupt
In service B |
54CD3210 |
5=gpip 5
4=gpip4 C=timer C D=timer D 3=gpip3 2=gpip2 1=gpip1 0=gpip 0 |
$e88013 |
MFP:Interrupt
Mask A |
76AFRETB |
7=Gpip7
6=Gpip6 A=Timer A F=recieve buffer Full R=Recieve error E=send
buffer Empty T=transmit error B=timer B |
$e88015 |
MFP:Interrupt
Mask B |
54CD3210 |
5=gpip 5
4=gpip4 C=timer C D=timer D 3=gpip3 2=gpip2 1=gpip1 0=gpip 0 |
$e88017 |
MFP:Interrupt
Vector register |
VVVVA--- |
V= Channel
0-16 handled automatically by MFP, A=All interrupts on (ISRA/B on) |
$e88019 |
MFP:Timer A
control register |
000T1000 |
T=Reset
signal 1000=Timer A event count mode |
$e8801B |
MFP:Timer B
control register |
000T1000 |
T=Reset
signal 1000=Timer B event count mode |
$e8801D |
MFP:Timer CD
control register |
0CCC0DDD |
C=Timer C
Delay mode / D=Timer D Delay mode |
$e8801F |
MFP:Timer A
Data Register |
|
|
$e88021 |
MFP:Timer B
Data Register |
|
|
$e88023 |
MFP:Timer C
Data Register |
|
|
$e88025 |
MFP:Timer D
Data Register |
|
|
$e88029 |
MFP:USART
Keyboard signal |
10000100D |
D= |
$e8802B |
MFP:Transmission
Status (Send) |
? |
|
$e8802D |
MFP:Transmission
Status (Recieve) |
|
|
Interrupt 6 - Gpip4 is the Vblank (referred to as V-Disp) controlled by
bit 7 of $E88009 and $E88015 - This causes an execution of vector $46 at
address $000118
The default vector base for the interrupts is vector $40 at address
$000100 - this can be changed with the top 4 bits of $e88017
Address |
Function |
Bits |
$000100 |
$40 |
UserInterrupt:
MFP RTC Alarm/1Hz |
$000104 |
$41 |
UserInterrupt:
MFP External power OFF |
$000118 |
$42 |
UserInterrupt:
MFP Front switch OFF |
$00010c |
$43 |
UserInterrupt:
MFP FM Audio source |
$000110 |
$44 |
UserInterrupt:
MFP Timer-D (Used with BG processing) |
$000114 |
$45 |
UserInterrupt:
MFP Timer-C (Mouse/cursor/FDD control, etc.) |
$000118 |
$46 |
UserInterrupt:
MFP V-DISP1(Vblank / GPIP4) |
$00011c |
$47 |
UserInterrupt:
MFP RTC Clock |
$000120 |
$48 |
UserInterrupt:
MFP Timer-B |
$000124 |
$49 |
UserInterrupt:
MFP Key serial output error |
$000128 |
$4a |
UserInterrupt:
MFP Key serial output empty |
$00012c |
$4b |
UserInterrupt:
MFP Key serial input error |
$000130 |
$4c |
UserInterrupt:
MFP Key serial input |
$000134 |
$4d |
UserInterrupt:
MFP Timer-A |
$000138 |
$4e |
UserInterrupt:
MFP CRTC*IRQ |
$00013c |
$4f |
UserInterrupt:
MFP H-SYNC |
$000140 |
$50 |
UserInterrupt:
SCC(B) Transmission buffer empty |
$000144 |
$51 |
UserInterrupt:
SCC(B) '' |
$000148 |
$52 |
UserInterrupt:
SCC(B) External/status changes |
$00014c |
$53 |
UserInterrupt:
SCC(B) '' |
$000150 |
$54 |
UserInterrupt:
SCC(B) Incoming character validity (Mouse 1 byte input) |
$000154 |
$55 |
UserInterrupt:
SCC(B) '' |
$000158 |
$56 |
UserInterrupt:
SCC(B) Special Rx condition |
$00015c |
$57 |
UserInterrupt:
SCC(B) '' |
$000160 |
$58 |
UserInterrupt:
SCC(A) Transmission buffer empty |
$000164 |
$59 |
UserInterrupt:
SCC(A) '' |
$000168 |
$5a |
UserInterrupt:
SCC(A) External status changes |
$00016c |
$5b |
UserInterrupt:
SCC(A) '' |
$000170 |
$5c |
UserInterrupt:
SCC(A) Incoming character validity (RS-232C 1 byte input) |
$000174 |
$5d |
UserInterrupt:
SCC(A) '' |
$000178 |
$5e |
UserInterrupt:
SCC(A) Special Rx Condition |
$00017c |
$5f |
UserInterrupt:
SCC(A) '' |
$000180 |
$60 |
UserInterrupt:
I/O FDC status interruption |
$000184 |
$61 |
UserInterrupt:
I/O FDC insertion/discharge interruption |
$000188 |
$62 |
UserInterrupt:
I/O HDC status interruption |
$00018c |
$63 |
UserInterrupt:
I/O Printer ready interruption |
$000190 |
$64 |
UserInterrupt:
DMAC #0 End (FDD) |
$000194 |
$65 |
UserInterrupt:
DMAC #0 Error ('') |
$000198 |
$66 |
UserInterrupt:
DMAC #1 End (SASI) |
$00019c |
$67 |
UserInterrupt:
DMAC #1 Error ('') |
$0001a0 |
$68 |
UserInterrupt:
DMAC #2 End (IOCS _DMAMOVE,_DMAMOV_A,_DMAMOV_L) |
$0001a4 |
$69 |
UserInterrupt:
DMAC #2 Error ('') |
$0001a8 |
$6a |
UserInterrupt:
DMAC #3 End (ADPCM) |
$0001ac |
$6b |
UserInterrupt:
DMAC #3 Error ('') |
$000200 |
$6c |
UserInterrupt:
SPC SCSI interruption (Internal SCSI) |
$000204 |
$6d |
UserInterrupt:
Unused |
$0003d4 |
$f5 |
UserInterrupt:
Unused |
$0003d8 |
$f6 |
UserInterrupt:
SPC SCSI interruption (SCSI board) |
$0003dc |
$f7 |
UserInterrupt:
Unused |
$0003fc |
$ff |
UserInterrupt:
Unused |
The
Xfile format
Executable files on the X68000 are in
'Xfile' format...
Vasm's linker cannot make this file, so we'll hhave to build it
ourselves, fortunately it's easy to create a simple xfile
we just need to add 64 bytes to the start of our program code,
&4855 ("HU" in ascii) at byte &00 and a 32 bit length in BIG
ENDIAN at byte 12
it sounds strage, but our code is considered the 'Text segment'...
we don't really need the other segments for our hello world type
example.
The Data segment
follows the code in the text segment, it's start is denoted by the
'.data' command in our asm code
After our 'Data Segment' there is a 'Relocation Table'... because we
don't know where our program will end up in memory, if we try to
load the address of part of the data we have, we don't know where it
will be...
If we use LEA (Load effective address) then we will get the correct
address, however if we do not use this command, then the address our
command uses will need to be corrected by the operating system, and
this is what the
Address relocation table does... it stores all the
addresses of the code that need to be fixed with the correct address
of the data as it appears after the xfile is loaded
The Symbol table
is our debugging infomation, it stores the addresses of the labels
in our code - we don't need it if we just want to run a program
the latest builds of VASM
(since 1.8e) can output directly to XFIle with the -Fxfile switch
|
addr |
bytes |
value |
notes |
$000000 |
2 |
$4855 |
Xfile id |
$000002 |
2 |
$0000 |
Reserved area |
$000004 |
4 |
Base Addr |
Linker's Base address |
$000008 |
4 |
Start addr |
Start address from Base
addr |
$00000C |
4 |
Text size |
Text segment size |
$000010 |
4 |
Data Size |
Data segment size |
$000014 |
4 |
Heap Size |
BSS+Stack |
$000018 |
4 |
RelocTblSz |
Address Relocation
Table size |
$00001C |
4 |
SymbTblSz |
Symbol table size |
$000020 |
32 |
$00 |
Reserved area |
$000040 |
TextSz |
|
Text Segment |
Offset1 |
DataSz |
|
Data Segment |
Offset2 |
RelocTblSz |
|
Address Relocation
Table |
Offset3 |
SymbTblSz |
|
Symbol Table |
Offset1=$40+TextSz
Offset2=Offset1+DataSz
Offset3=Offset2+RelocTblSz
|
The Xfile format - broken
down
We're going to use the official x68000 assembler (on an emulator)
to compile an ASM file, and see the result in the binary Xfile...
The example to the right doesn't really do anything, it's just got
some different contents, which should be easy to see in the
resulting output.
There is a code (text) segment, a data segment, and some symbols
We'll compile it with AS, and look what happens! |
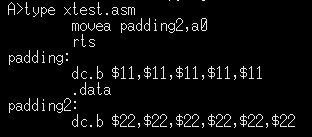 |
The Assembler will produce this
output if we use the -p option to output the listing... we've also
included -d to tell it to output the symbols to the binary file.
|
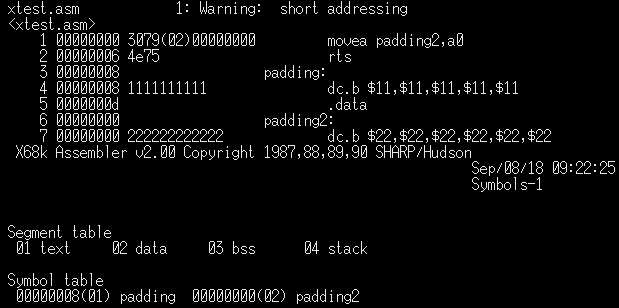 |
Once we've used LK to convert the object file to a X file, we can
see the result.
The binary file is shown to the right, we can see the following
sections...
The Header
is at the start of the file
The Code
(text) segment - it's
size
can be seen in the header
The Data
segment -
it's size is also in the
header
The Symbol
table - it's
size
is also in the header.
The
Relocation table has the addresses of code which needs it's
addresses altering - it's
size
is also in the header |
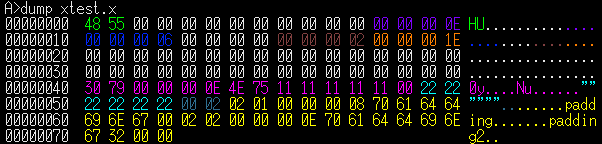 |
Supervisor mode and
XM6
On WinX68kHighSpeed we can access hardware registers in USER mode,
but emulator XM6 is more strict.
We will need to enter 'Supervisor mode'... we do this with DOSCALL
function _SUPER ($FF20)...
Thanks to viewer 'Mugsy' for this info |
|
The Symbol table
The symbol
table is made up of a series of entries in the format shown below.
Address |
Bytes |
Function |
$00 |
2 |
Type (see table to right) |
$02 |
4 |
value |
$06 |
?? |
Zero Terminated String (rounded to even byte) |
|
Type |
Format of
symbol |
$0003 |
common rcommon rlcommon |
$0200 |
absolute rdata rbss rstack rldata rlbss rlstack |
$0201 |
text |
$0202 |
data |
$0203 |
bss |
$0204 |
stack |
|
Using the Sharp/Hudson x68k assembler
The X68000's assembler is pretty easy to use, in fact it may be easier than
the PC ones! to compile prog.asm we just type:
AS prog.asm
LK prog.o
This will output an file prog.x ... which you can run from the command line
A more advanced use of AS is:
AS -p prog.lst -d prog.asm
-p file.name will save the listing
to a text file
-d will include debugging
symbols in the output
You can get the assembler here
Using
ED.X - the x68k text editor
You may wish to do your assembly
programming on the x68 itself, but even if you don't you may need to
edit batch files and settings files on your x68 emulator.
Fortunately, the x68 has a built in editor called ED.X - it's pretty
easy to use, but you'll need to know the basic keypresses to save
and load your work. the most useful keypresses are show to the
right.
A full translated copy of the Edit help is shown below |
Keypress |
Command |
ESC-E |
Save All files and Exit |
ESC-T |
Rename file |
ESC-X |
Save and exit |
ESC-Q |
Don't save and Quit |
ESC-O |
Reset to last save |
|
ED.HLP translated
contents
|
|
|
|
CTRLキー 機能一覧 |
CTRL Key Command List |
CTRL+A |
カーソルを1語後方(←)に移動 |
Move the cursor to one word (←) |
CTRL+B |
カーソルを行の左端(または右端)に移動 |
Move the cursor to the left end (or right end) of the
line |
CTRL+C |
画面をロールアップ |
Roll up the screen |
CTRL+D |
カーソルを1文字右に移動 |
Move the cursor to the right |
CTRL+E |
カーソルを1行上に移動 |
Move the cursor to one line |
CTRL+F |
カーソルを1語前方(→)に移動 |
Move the cursor to one word (→) |
CTRL+G |
1文字削除 |
Remove one letter |
CTRL+H |
バックスペース |
Backspace |
CTRL+I |
水平タブ |
Horizontal tab |
CTRL+J |
ヘルプ画面の表示 |
Help screen display |
CTRL+K |
カーソル位置から行末までの削除 |
Remove from cursor position to end |
CTRL+L |
削除文字列バッファの内容を挿入 |
Insert the contents of the delete string buffer |
CTRL+M |
改行と行分割(行分割はインサート状態の時のみ) |
Line breaks and row division (line division only when
insert state) |
CTRL+N |
カーソル行の上に1行挿入 |
1 line inserted on the cursor line |
CTRL+O |
挿入ON/OFF |
Insertion ON / OFF |
CTRL+P |
カーソルを右端に移動 |
Move the cursor to the right edge |
CTRL+Q |
カーソルを左端に移動 |
Move the cursor to the left |
CTRL+R |
画面をロールダウン |
Roll down the screen |
CTRL+S |
カーソルを1文字左に移動 |
Move the cursor to the left one character |
CTRL+T |
1語削除 |
1 word delete |
CTRL+U |
行頭からカーソルの直前までを削除 |
Remove from the beginning to just before the cursor |
CTRL+V |
コントロールコードの入力 |
Control code input |
CTRL+W |
画面を1行分ロールダウン |
Roll down one line of screen |
CTRL+X |
カーソルを1行下に移動 |
Move the cursor down one row |
CTRL+Y |
1行削除 ---->削除文字列バッファへ移動 |
1 row delete ---> Move to delete string buffer |
CTRL+Z |
画面を1行分ロールアップ |
Roll up one line of screen |
CTRL+[ |
ESCコマンド |
ESC command |
CTRL+\ |
カレントワード後方検索 |
Current word after retrieval search |
CTRL+] |
大文字・小文字変換 |
Uppercase and lower case conversion |
CTRL+^ |
カレントワード前方検索 |
Current word forward search |
CTRL+_ |
ファイル終端記号 |
File termination symbol |
|
|
|
|
ESC コマンド一覧 |
ESC Command List |
(ESC+@) |
キーボードマクロの定義 |
Keyboard macro definition |
(ESC+A) |
ファィルの切り替え(昇順) |
File switching (ascending order) |
(ESC+B) |
ファィルの先頭 |
First of the File |
(ESC+C) |
子プロセスの実行 |
Run child process |
(ESC+D) |
ファィルの切り替え(降順) |
File switching (descending order) |
(ESC+E) |
編集中の全部のテキストをセーブしエディタを終了する |
Save all text while editing and exit the editor |
(ESC+F) |
新しいファイルの編集 |
Edit new file |
(ESC+nG) |
カットバッッファの内容をカーソル位置にn回複写 |
N times in the cursor position of the contents of the
cut buffer |
(ESC+H) |
現在の編集テキストをセーブする。編集は継続 |
Save the current edit text. Edit continues |
(ESC+I) |
タブ文字の表示/非表示 |
View / Hide Tab Characters |
(ESC+J) |
文字列の連続置換(前方) 確認あり |
Continuous replacement of strings (forward)
confirmation |
(ESC+K) |
現在の編集テキストをセーブせずに編集を終了する |
End editing without saving the current edit text |
(ESC+L) |
文字列の連続置換(後方) 確認あり |
Continuous replacement of strings (rear) confirmation |
(ESC+M) |
改行文字の表示/非表示 |
Show / hide newline characters |
(ESC+N) |
前方検索 |
Forward search |
(ESC+O) |
現在の編集テキストの編集を最初からやり直す |
Return the editing of the current edit text from the
beginning |
(ESC+nP) |
カーソル位置からn行カットバッファに移動 |
Move from cursor position to n line cut buffer |
(ESC+Q) |
テキストをセーブせずに強制的にエディタを終了する |
Forced to end the editor without saving the text |
(ESC+R) |
文字列の連続置換(前方) 確認なし |
Continuous replacement of strings (forward) without
confirmation |
(ESC+S) |
後方検索 |
Rear search |
(ESC+T) |
現在の編集テキストのファィル名を変更する |
Change File Name of Current Edit Text |
(ESC+U) |
文字列の連続置換(後方) 確認なし |
Continuous replacement of strings (rear) no
confirmation |
(ESC+V) |
タグジャンプ |
Tag jump |
(ESC+W) |
ファィルの書き出し |
File export |
(ESC+X) |
現テキストのセーブと編集の終了 |
Current text save and edit end |
(ESC+Y) |
ファィルの読み込み |
File loading |
(ESC+Z) |
ファィル最終行 |
File last line |
(ESC+[) |
カレント前方置換(確認なし) |
Current front substitution (no confirmation) |
(ESC+\) |
カレント後方置換(確認なし) |
Current rear replacement (without confirmation) |
(ESC+]) |
大文字/小文字変換 |
Uppercase / lower case conversion |
(ESC+^) |
前方置換(表示なし) |
Forward replacement (without display) |
(ESC+_) |
後方置換(表示なし) |
Backward replacement (without display) |
(ESC+NUM) |
指定行番号へのジャンプ |
Jump to the specified number |
|
|
|
|
ファンクションキー 機能一覧 |
Function key function list |
F-1 |
ファィルの先頭 |
First of the File |
F-2 |
ファィル最終行 |
File last line |
F-3 |
文字列の連続置換(確認なし)(前方) |
Continuous replacement of strings (no confirmation)
(forward) |
F-4 |
文字列の前方↓検索 |
Predemary of strings ↓ search |
F-5 |
カレント検索文字列の前方↓検索 |
Foreign front of the current search string |
F-6 |
行単位でのテキストの選択操作を開始(範囲) |
Starting the text selection operation in row units
(range) |
F-7 |
選択したテキストをカットバッファへ移動する |
Move the selected text to the cut buffer |
F-8 |
選択したテキストをカットバッファへ複写する |
Copy the selected text to the cut buffer |
F-9 |
行カットバッファの内容をカーソル行の上に挿入 |
Insert the contents of the row cut buffer onto the
cursor line |
F-10 |
行の二重化 |
F-9 Insert the contents of the line cut buffer onto
the cursor line |
SHIFT F-1 |
新編集 |
New edit |
SHIFT F-2 |
再編集 |
re-edit |
SHIFT F-3 |
文字列の連続置換(確認なし)(後方) |
Continuous replacement of strings (without
confirmation) (backward) |
SHIFT F-4 |
文字列の後方↑検索 |
Backward search for strings |
SHIFT F-5 |
カレント検索文字列の後方↑検索 |
Backward ↑ search for the current search string |
SHIFT F-6 |
ファィルの切り替え(昇順) |
File switching (ascending order) |
SHIFT F-7 |
ファィルの切り替え(降順) |
File switching (descending order) |
SHIFT F-8 |
ファィルの読み込み |
File loading |
SHIFT F-9 |
ファィルの書き出し |
File export |
SHIFT F-10
|
子プロセスの実行 |
Run child process
|
|
|
|
|
単一キー 機能一覧 |
Single key function list |
ROLLUP |
画面をロールアップ |
Roll up the screen |
ROLLDWN |
画面をロールダウン |
Roll down the screen |
CR |
改行と行分割(行分割はインサート状態の時のみ) |
Line breaks and row division (line division only when
insert state) |
TAB |
水平タブ |
Horizontal tab |
BS |
バックスペース |
Backspace |
INS |
挿入MODE ON /OFF |
Insertion MODE ON / OFF |
DEL |
1文字削除 |
Remove one letter |
CLR |
ファィル終了記号の表示 |
Display File End Sign |
HELP |
ヘルプ画面の表示 |
Help screen display |
HOME |
ホーム位置に移動 |
Move to home position |
LEFT |
カーソルを左に移動 |
Move the cursor to the left |
RIGHT |
カーソルを右に移動 |
Move the cursor to the right |
UP |
カーソルを上に移動 |
Move the cursor up |
DOWN |
カーソルを下に移動 |
Move the cursor down |
ESC |
ESCコマンド |
ESC command |
UNDO |
キーボードマクロの実行 |
Running a keyboard macro |
|
|
|
|
起動時のオプション |
Startup option |
-A |
ヘルプファイルのパス指定 |
Help file path specification |
-A |
デフォルトは ed.x のあるパス |
The default is the path with ed.x |
-B |
テキストの終わりを物理的なデータの終わりで認識 |
Recognize the end of the text at the end of physical
data |
-B |
することを指定する |
Specify that |
|
デフォルトは テキストファイルの終わりを
EOF(&H1A) |
The default is EOF (& H1A) the end of the text
file |
|
コードで認識します |
Recognize in code |
-E |
ファィル終了記号の表示を指定します |
Specifies the display of the file end symbol |
-H |
水平タブの最大表示幅を指定します ( 2 / 4 / 6 /
8 ) |
Specifies the maximum display width of the horizontal
tab (2/4/6 / 8) |
-L |
改行文字の表示を指定します |
Specify the display of newline characters |
-L |
デフォルトは 表示しません |
Default does not display |
-M |
一行の長さを指定します |
Specify a line length |
-M |
(128/256/512/1024 BYTES) |
(128/256/512/1024 bytes) |
-S |
画面サイズを指定します |
Specify the screen size |
-S |
1 96(XPOS)*30 |
1 96 (XPOS) * 30 |
|
2 64(XPOS)*30 |
2 64 (XPOS) * 30 |
-T |
タブ文字の表示を指定します |
Specify the display of tab characters |
X68000 Links!
Replacing an
x68000 powersupply - The power supply breaks a lot, but can easilly be
replaced with a PC Pico-ATX psu!
Connecting a HxC to the x68000 - Note,
while this somewhat works, there are problems due to the extra control lines
not being connected
External
floppy pinout - I used this to connect my HxC to my x68000 and create
disks from images
Connecting
SCSI2SD
to the x68000 - a cheap SD emulator for the SCSI/SASI hard drive
| |
Buy my Assembly programming book on Amazon in Print or Kindle!
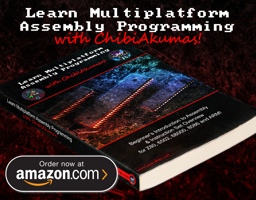
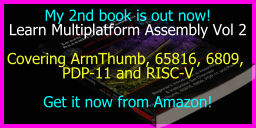
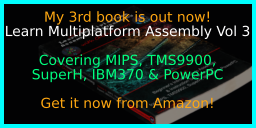
Available worldwide! Search 'ChibiAkumas' on your local Amazon website!
Click here for more info!
|